Bootstrap 4 carousel is a slideshow component that slides images and text in a cyclic manner. The carousel may also contain previous and next controls and indicators to click and move the content in a slideshow. It is useful when you want to display large-size content in a small area.
Let’s find out how to create a carousel with the example given below.
Simple Slide Only Carousel in Bootstrap 4
A simple slide is a carousel that contains images that are slide automatically in a cyclic manner. To create a carousel, you need to add the class .carousel
and .slide
with data-attribute="carousel"
to the <div>
element. Inside that <div>
, place the class .carousel-inner
inside which each image items is located within the class .carousel-item
individually.
It is also required to add the .active
class to any one of the class .carousel-item
as given below:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
<div id="carousel-demo" class="carousel slide" data-ride="carousel"> <!-- Carousel Items --> <div class="carousel-inner"> <div class="carousel-item active"> <img src="https://tutorialdeep.com/images/slide-img1.png" alt=""> </div> <div class="carousel-item"> <img src="https://tutorialdeep.com/images/slide-img2.png" alt=""> </div> <div class="carousel-item"> <img src="https://tutorialdeep.com/images/slide-img3.png" class="" alt=""> </div> </div> </div> |
Output
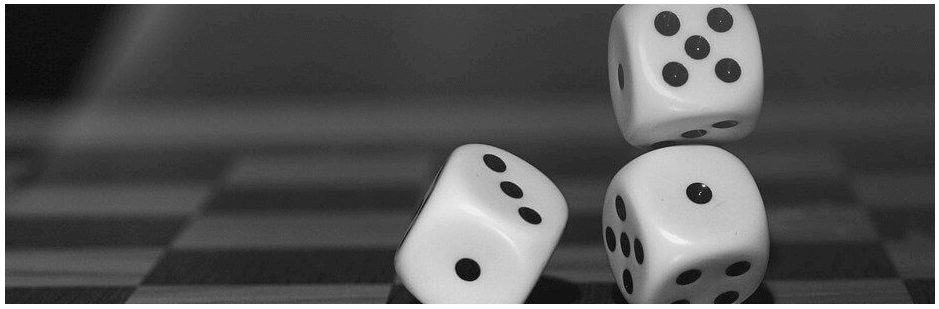
The above example contains three images that slide automatically.
Carousel with Controls and Indicators
Controls and indicators in a carousel are useful to click and move the slide content. Indicators are useful to check the location of the slideshow and the present sliding image in a carousel.
How to Create Indicators
To create an indicator, you need to add the number of list items same as the number of items in a carousel with class .carousel-indicators
to the <ol>
element. After that, add an id
attribute to the <div>
element that contains the .carousel
class.
Add the attribute data-target="#id"
to all the indicator list items and replace the id with the id of the carousel. Also, add another attribute data-slide-to
to the indicator list items with index value starts from zero(0).
1 2 3 4 5 6 |
<!-- Carousel Indicators --> <ol class="carousel-indicators"> <li data-target="#carousel-demo" data-slide-to="0" class="active"></li> <li data-target="#carousel-demo" data-slide-to="1"></li> <li data-target="#carousel-demo" data-slide-to="2"></li> </ol> |
Create Controls in a Carousel
To create controls, you need to add the class .carousel-control-prev
and attribute data-slide="prev"
to the <a>
element with #id
(The #id
is the id of the carousel) in the href
attribute. Inside that <a>
element, place the class .carousel-control-prev-icon
to the <span>
element to create the previous icon.
Similarly, you can create the next control by placing the next
in place of prev
as given below:
1 2 3 4 5 6 7 |
<!-- Carousel Left and Right Controls --> <a href="#carousel-demo" class="carousel-control-prev" data-slide="prev"> <span class="carousel-control-prev-icon"></span> </a> <a href="#carousel-demo" class="carousel-control-next" data-slide="next"> <span class="carousel-control-next-icon"></span> </a> |
Complete Carousel Code with Controls and Indicators
The complete code to create a carousel with controls and indicators is given below:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
<div id="carousel-demo" class="carousel slide" data-ride="carousel"> <!-- Carousel Indicators --> <ol class="carousel-indicators"> <li data-target="#carousel-demo" data-slide-to="0" class="active"></li> <li data-target="#carousel-demo" data-slide-to="1"></li> <li data-target="#carousel-demo" data-slide-to="2"></li> </ol> <!-- Carousel Items --> <div class="carousel-inner"> <div class="carousel-item active"> <img src="https://tutorialdeep.com/images/slide-img1.png" alt=""> </div> <div class="carousel-item"> <img src="https://tutorialdeep.com/images/slide-img2.png" alt=""> </div> <div class="carousel-item"> <img src="https://tutorialdeep.com/images/slide-img3.png" class="" alt=""> </div> </div> <!-- Carousel Left and Right Controls --> <a href="#carousel-demo" class="carousel-control-prev" data-slide="prev"> <span class="carousel-control-prev-icon"></span> </a> <a href="#carousel-demo" class="carousel-control-next" data-slide="next"> <span class="carousel-control-next-icon"></span> </a> </div> |
Output
Create Carousel with Captions in Bootstrap 4
A caption is content you can place at the top of each image. You can create captions for all the images by adding them to the class .carousel-caption
and wrap it inside the class .carousel-item
as given below:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 |
<div id="carousel-demo" class="carousel slide" data-ride="carousel"> <!-- Carousel Indicators --> <ol class="carousel-indicators"> <li data-target="#carousel-demo" data-slide-to="0" class="active"></li> <li data-target="#carousel-demo" data-slide-to="1"></li> <li data-target="#carousel-demo" data-slide-to="2"></li> </ol> <!-- Carousel Items --> <div class="carousel-inner"> <div class="carousel-item active"> <img src="https://tutorialdeep.com/images/slide-img1.png" alt=""> <div class="carousel-caption"> <h5>First slide label</h5> <p>First slide content</p> </div> </div> <div class="carousel-item"> <img src="https://tutorialdeep.com/images/slide-img2.png" alt=""> <div class="carousel-caption"> <h5>Second slide label</h5> <p>Second slide content</p> </div> </div> <div class="carousel-item"> <img src="https://tutorialdeep.com/images/slide-img3.png" class="" alt=""> <div class="carousel-caption"> <h5>Third slide label</h5> <p>Third slide content</p> </div> </div> </div> <!-- Carousel Left and Right Controls --> <a href="#carousel-demo" class="carousel-control-prev" data-slide="prev"> <span class="carousel-control-prev-icon"></span> </a> <a href="#carousel-demo" class="carousel-control-next" data-slide="next"> <span class="carousel-control-next-icon"></span> </a> </div> |
Output
Fade Animated Carousel Slider in Bootstrap 4
The default behavior of the carousel is to slide the images and text content in a cyclic manner. However, you can change the sliding behavior of the carousel by adding the class .carousel-fade
to the <div>
element that contains the class .carousel
and .slide
as given below:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
<div id="carousel-demo" class="carousel slide carousel-fade" data-ride="carousel"> <!-- Carousel Items --> <div class="carousel-inner"> <div class="carousel-item active"> <img src="https://tutorialdeep.com/images/slide-img1.png" alt=""> </div> <div class="carousel-item"> <img src="https://tutorialdeep.com/images/slide-img2.png" alt=""> </div> <div class="carousel-item"> <img src="https://tutorialdeep.com/images/slide-img3.png" class="" alt=""> </div> </div> <!-- Carousel Left and Right Controls --> <a href="#carousel-demo" class="carousel-control-prev" data-slide="prev"> <span class="carousel-control-prev-icon"></span> </a> <a href="#carousel-demo" class="carousel-control-next" data-slide="next"> <span class="carousel-control-next-icon"></span> </a> </div> |
Output
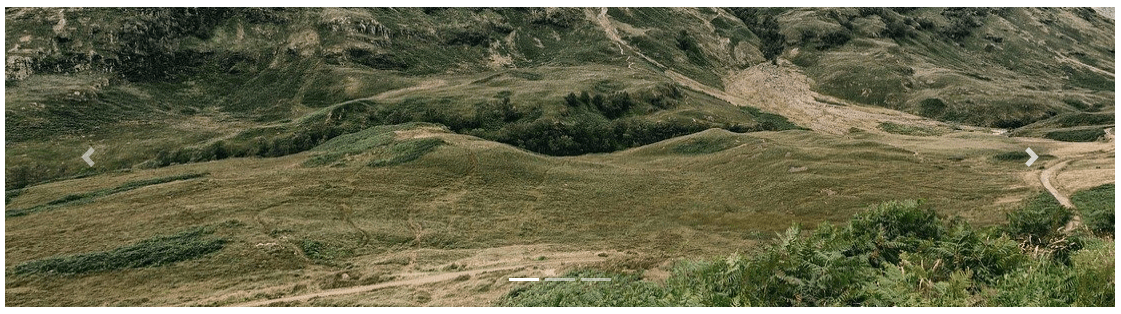
Time Delay To Slide Between Items
You can delay the sliding images in a carousel by adding the attribute data-interval
to the <div>
element that contains the class .carousel-item
. The value of the attribute is the time in a millisecond and you can add the attribute to each image in a carousel for the time delay.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
<div id="carousel-demo" class="carousel slide" data-ride="carousel"> <!-- Carousel Items --> <div class="carousel-inner"> <div class="carousel-item active" data-interval="12000"> <img src="https://tutorialdeep.com/images/slide-img2.png" alt=""> </div> <div class="carousel-item" data-interval="3000"> <img src="https://tutorialdeep.com/images/slide-img1.png" alt=""> </div> <div class="carousel-item"> <img src="https://tutorialdeep.com/images/slide-img3.png" class="" alt=""> </div> </div> <!-- Carousel Left and Right Controls --> <a href="#carousel-demo" class="carousel-control-prev" data-slide="prev"> <span class="carousel-control-prev-icon"></span> </a> <a href="#carousel-demo" class="carousel-control-next" data-slide="next"> <span class="carousel-control-next-icon"></span> </a> </div> |
Output
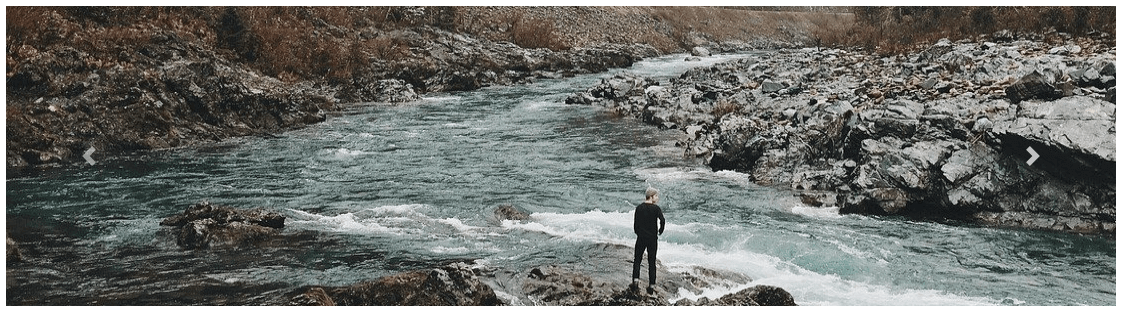