Learn how to add datepicker in Bootstrap 5 and other versions. The short answer is to use the Flatpickr lightweight library. let’s find out some examples.
Add Datepicker in Bootstrap 5
To add datepicker in Bootstrap 5, you can use the flatpickr lightweight library. It is highly customize and works great with Bootstrap as given below.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
<!-- Flatpickr CSS --> <link href="https://cdn.jsdelivr.net/npm/flatpickr/dist/flatpickr.min.css" rel="stylesheet"> <!-- Bootstrap Icons (Optional) --> <link href="https://cdn.jsdelivr.net/npm/bootstrap-icons/font/bootstrap-icons.css" rel="stylesheet"> <label for="datepicker" class="form-label">Select a Date</label> <div class="input-group"> <input type="text" id="datepicker" class="form-control" placeholder="Select a date"> <span class="input-group-text"> <i class="bi bi-calendar"></i> </span> </div> <!-- Flatpickr JS --> <script src="https://cdn.jsdelivr.net/npm/flatpickr"></script> <script> document.addEventListener('DOMContentLoaded', function () { flatpickr("#datepicker", { dateFormat: "Y-m-d", // Adjust the format as needed (e.g., Y-m-d, d-m-Y, etc.) allowInput: true // Allows manual input }); // Open datepicker on icon click document.querySelector("#calendar-icon").addEventListener("click", function () { datepicker.open(); }); }); </script> |
Output
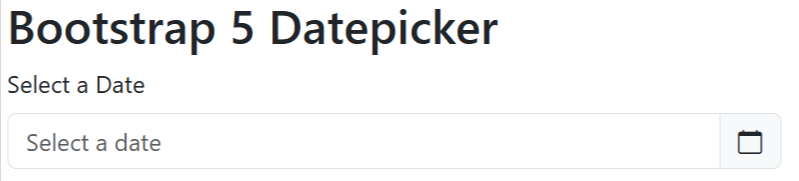
Click the input field given above to see the live preview of the calendar. The calendar is easy to use and select months and years. It also uses the Bootstrap icons library to display the calendar icon with input field.
Create Datepicker in Bootstrap 4
If you want to create a datepicker in Bootstrap 4, you can also use the Flatpickr library. See the example below to learn the method.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
<!-- Flatpickr CSS --> <link href="https://cdn.jsdelivr.net/npm/flatpickr/dist/flatpickr.min.css" rel="stylesheet"> <!-- Bootstrap Icons (Optional) --> <link href="https://cdn.jsdelivr.net/npm/bootstrap-icons/font/bootstrap-icons.css" rel="stylesheet"> <label for="datepicker" class="form-label">Select a Date</label> <div class="input-group"> <input type="text" id="datepicker" class="form-control" placeholder="Select a date"> <div class="input-group-append"> <span class="input-group-text" id="calendar-icon"> <i class="bi bi-calendar"></i> <!-- Calendar icon --> </span> </div> </div> <!-- Flatpickr JS --> <script src="https://cdn.jsdelivr.net/npm/flatpickr"></script> <script> document.addEventListener('DOMContentLoaded', function () { flatpickr("#datepicker", { dateFormat: "Y-m-d", allowInput: true }); // Open datepicker on icon click document.querySelector("#calendar-icon").addEventListener("click", function () { datepicker.open(); }); }); </script> |
Output
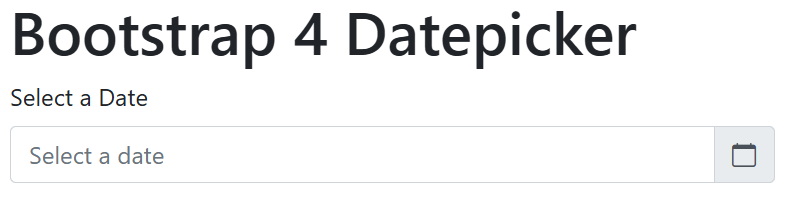
You can click the input field to see the live preview with datepicker calendar.
It uses the same calendar icon with the input field. However, Bootstrap 4 requires to add extra div with class .input-group-append
and .input-group-text
to group the calendar with input field.
Date Picker in Bootstrap 3
In Bootstrap 3, you can also use the flatpickr. But, it require a jQuery library to include and work as given below.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
<!-- Flatpickr CSS --> <link href="https://cdn.jsdelivr.net/npm/flatpickr/dist/flatpickr.min.css" rel="stylesheet"> <!-- Bootstrap Icons (Optional) --> <link href="https://cdn.jsdelivr.net/npm/bootstrap-icons/font/bootstrap-icons.css" rel="stylesheet"> <label for="datepicker" class="control-label">Select a Date</label> <div class="input-group"> <input type="text" id="datepicker" class="form-control" placeholder="Select a date"> <span class="input-group-addon" id="calendar-icon"> <i class="bi bi-calendar"></i> <!-- Calendar icon --> </span> </div> <!-- Flatpickr JS --> <script src="https://cdn.jsdelivr.net/npm/flatpickr"></script> <script> $(document).ready(function () { // Initialize Flatpickr on the input field const datepicker = flatpickr("#datepicker", { dateFormat: "Y-m-d", // Customize the date format allowInput: true, // Allows users to manually type dates }); // Open Flatpickr on calendar icon click $("#calendar-icon").on("click", function () { datepicker.open(); // Open Flatpickr programmatically }); }); </script> |
Output
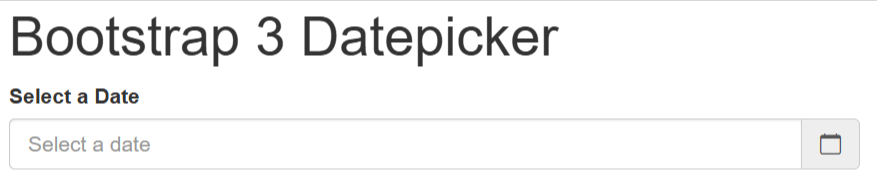
When you click the input field given above, you will get the live preview where you will get the calendar to select the date. For combining the calendar icon with the input field, you have to use the class .input-group-addon
.
Bootstrap 3 does not work with Javascript and require a latest jQuery library to work with the Flatpickr.